Adding a Stylish Loader to Your Website
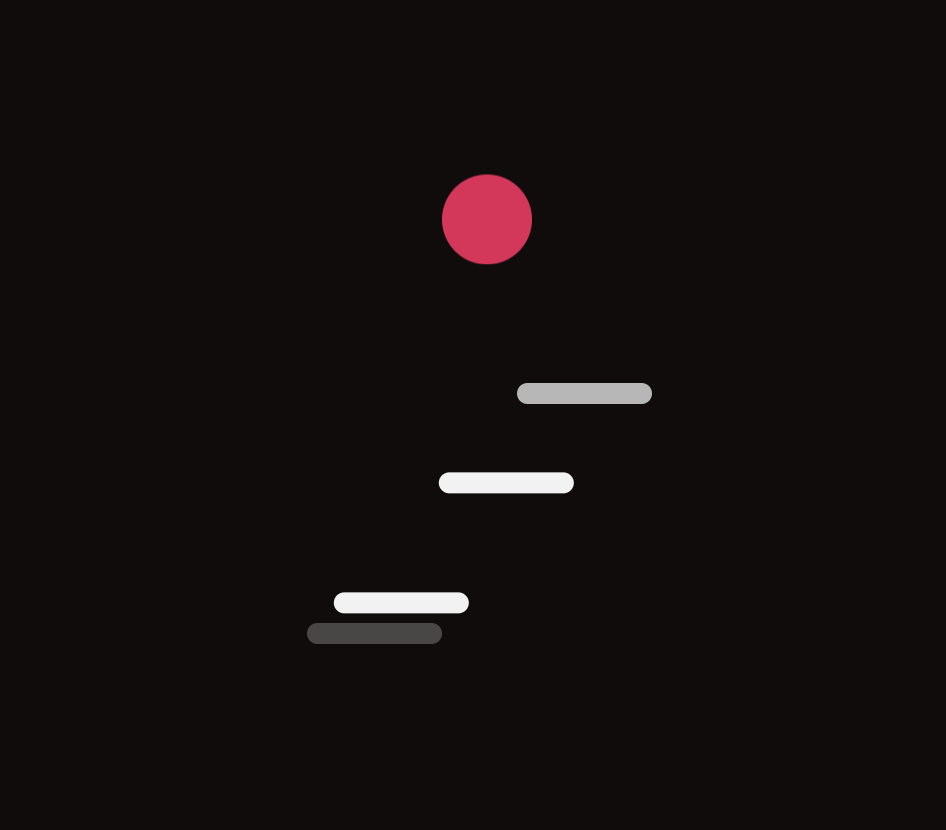
As web developers, we often need to implement loading indicators to provide visual feedback to users while content is being fetched or processed. In this tutorial, we'll explore how to create an engaging loader animation using React and Tailwind CSS.
Introduction
A well-designed loader can significantly enhance the user experience of your web application. It not only informs users that something is happening behind the scenes but also adds a touch of interactivity and polish to your site. The loader we'll be creating features a bouncing ball animation, which is both visually appealing and informative.
Setting up the Loader Component
Let's start by creating our Loader component. Here's the React code for our loader:
Code Snippet
This component creates a container with a bouncing ball and animated steps. The ball uses Tailwind's built-in animate-bounce
class, while the steps use a custom animation.
Adding Custom Animations
To make our loader work, we need to add some custom animations to our Tailwind configuration. Add the following to your tailwind.config.js
file:
module.exports = {
// ... other configurations
theme: {
extend: {
animation: {
bounce: "loading-bounce 0.5s ease-in-out infinite alternate",
"loading-step": "loading-step 1s ease-in-out infinite",
},
keyframes: {
"loading-bounce": {
"0%": { transform: "scale(1, 0.7)" },
"40%": { transform: "scale(0.8, 1.2)" },
"60%": { transform: "scale(1, 1)" },
"100%": { bottom: "140px" },
},
"loading-step": {
"0%": {
boxShadow:
"0 10px 0 rgba(0, 0, 0, 0), 0 10px 0 #f2f2f2, -35px 50px 0 #f2f2f2, -70px 90px 0 #f2f2f2",
},
"100%": {
boxShadow:
"0 10px 0 #f2f2f2, -35px 50px 0 #f2f2f2, -70px 90px 0 #f2f2f2, -70px 90px 0 rgba(0, 0, 0, 0)",
},
},
},
},
},
// ... other configurations
};
These custom animations create the bouncing effect for the ball and the stepping motion for the platform.
Using the Loader
To use the loader in your application, you can simply import and render it where needed. For example:
import React, { useState, useEffect } from 'react';
import Loader from './Loader';
const MyComponent = () => {
const [isLoading, setIsLoading] = useState(true);
useEffect(() => {
// Simulate an API call
setTimeout(() => {
setIsLoading(false);
}, 3000);
}, []);
if (isLoading) {
return <Loader />;
}
return (
<div>
{/* Your component content */}
</div>
);
};
export default MyComponent;
In this example, we're using the Loader component while the isLoading
state is true. Once the loading is complete (simulated by a setTimeout), the actual component content is rendered.
Customizing the Loader
You can easily customize the loader by adjusting the CSS classes in the Loader component. For example, to change the color of the bouncing ball, you can modify the bg-primary
class to any other Tailwind color class or your custom color.
Conclusion
Adding a stylish loader to your website can greatly enhance the user experience by providing visual feedback during loading processes. With React and Tailwind CSS, you can create engaging animations that are both functional and aesthetically pleasing.
Remember to use loaders judiciously - they're most effective when used for operations that take a noticeable amount of time to complete. For near-instantaneous operations, a loader might not be necessary and could potentially slow down the perceived performance of your application.
By following this tutorial, you've learned how to create and implement a custom loader animation in your React application using Tailwind CSS. Feel free to experiment with different styles and animations to create a loader that fits perfectly with your website's design.